Ruby is an excellent programming language that is as simple as JavaScript and as versatile as C++.
Yukihiro Matsumoto, the author of Ruby, wanted to create the perfect object-oriented language to make the OOP approach work as fully as possible. Besides that, the new language should follow a set of rules that Yukihiro himself adhered to and which are now called “The Ruby Way”. Here are some of them:
A language for humans, not for computers – the program should be easy to read even with a minimal knowledge of Ruby, and the computer should do the rest.
Simple, but not too simple – everything should be simple in the program, but only if it does not require complicating something else.
The principle of least surprise – all commands and actions in Ruby should work exactly as the programmer expects them to. If he encounters something new in the language, it should not surprise him, on the contrary, he will take it as something natural.
“You don’t have to fight it” – the programmer should use what’s in the language instead of trying to tweak the language to suit himself.
The first version of Ruby came out in 1995, and in that time the language has grown from a one-man project to a large community and 1.27 million lines of code. Ruby is now a universal cross-platform programming language and is supported by all modern versions of Linux and MacOS.
Specificity of language
The beauty and power of Ruby is that everything in Ruby is an object. Even numbers, variable values and data types are objects. For example, you have addition, which is implemented like this:
“x = 7 + 5”
7 and 5 are numbers that the Numeric class is responsible for, and this class knows about the addition sign.
Let’s extend this class a little further:
“class Numeric
def plus(x)
self + (x)
end
end
y = 7.plus 5”
From this point, all numbers have a plus method that adds that number to the second number. And in the same way, you can extend the properties and capabilities of each element in the program.
And you can do this with anything, without restrictions and incompatibility of types. And how can there be incompatibilities when types are also objects that can be changed. This makes Ruby similar to C++, with operator overloading and the ability to use commands differently than originally intended.
Syntax
Ruby’s first priority is readability and simplicity, so it’s realistic to learn this language in a week at a basic level.
Notable things are that you don’t have to put a semicolon at the end of each command and all nesting is indicated by spaces, just like in Python.
Comments. We write a grid sign # at the beginning of the line, followed by a comment of any length.
Assignment and mathematical operators. This is simple: assignment is an equal sign “=”, and mathematical operators are the same as in life – +, -, *, etc.
Data types. Since everything in Ruby is an object, even data types are also objects, meaning you can change their properties and behavior. For this reason, there are very few built-in data types in Ruby. If a programmer needs something more complex than the built-in types, they can easily create their own type from scratch or based on the built-in type.
Whole numbers: Fixnum (up to 2³⁰) and Bignum. The second type can store integers of any size at all, as long as there is enough space for them in RAM.
Fractional numbers: Float (floating point numbers), Rational (rational numbers) and Complex (complex numbers with an imaginary unit).
String: String, a sequence of bytes in UTF-8 encoding, can be of any length.
A character in Ruby is not a single character, but just any string that stays the same. To let the computer know it’s a character, it’s preceded by a colon: symbol = :this_also_character.
Range – this is the responsibility of the Range class and stores a continuous sequence of integers:
- d1 = 1…5 # Two points – 1 to 5 inclusive.
- d2 = 1…5 # Three points – 1 to 4, and 5 is no longer in this range.
Loops. There are seven kinds of loops in Ruby, because you can swap out the beginning of a loop and its exit conditions, add counters or a forced stop statement.
Classes, objects and methods. Since Ruby is a fully object-oriented language, everything in the OOP(object-oriented programming) works in it:
- public and private methods,
- constructors,
- inheritance (with built-in extensions),
- class overrides,
- and anything else you need to do with objects or classes.
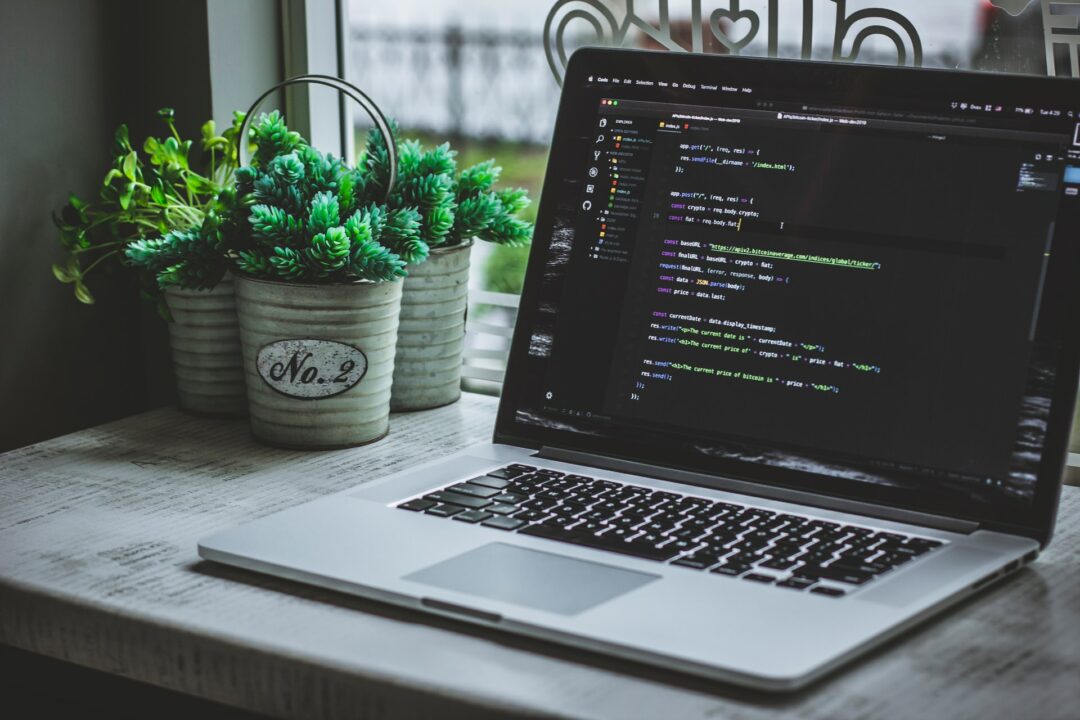
Why Ruby is needed?
Since Ruby is a scripting language, it can be used in any field of development. For example, you can write a parser pages, which will find on the site online shop all the product cards and download their names, prices and photos. Or you can make a generator of complex passwords for all visitors to the site, so they do not have to think it up yourself.
Mobile games and applications for Android and iOS are also written in Ruby, using Ruboto or RubyMotion.
But Ruby works best when paired with its famous Ruby on Rails web application framework. It allows you to quickly create a site with a complex structure, dynamic pages, and without having to learn SQL queries and work with databases.
The platform is also favoured by start-ups because it can be used to deploy a prototype project in a short time and immediately see its strengths and weaknesses.
What’s made in Ruby?
Ruby is used by many different levels of companies, including NASA, Motorola, Google or GitHub. Here are some of the projects that are written in Ruby or Ruby on Rails:What software does NASA use
- Twitter,
- Kickstarter,
- SoundCloud,
- Redmine (bug tracking system),
- Inkscape (vector graphics),
- GitLab (code repository management),
- SketchUp (three-dimensional modeling),
- Netflix (TV series),
- Bloomberg (news agency).
Looking at this list, it is clear that Ruby is especially good at web development and building sites with complex structures.
Comments are closed